矩阵乘法计算过程
编辑
12
2025-01-15
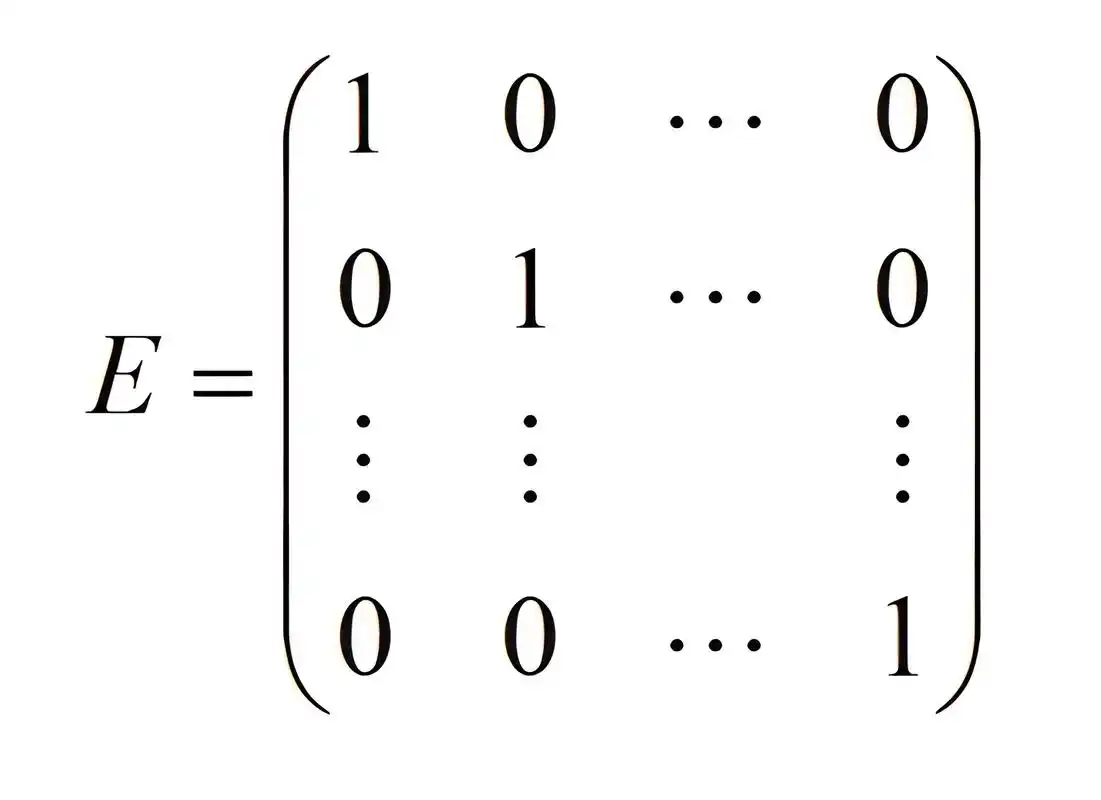
背景
最近看到python工具库numpy矩阵计算中,之前也有点迷惑的过程。重新使用python代码实现计算的过程,就给他记录下来了
计算过程
# 矩阵乘法
import numpy as np
a = np.arange(20).reshape(4,5)
a = np.asmatrix(a)
print(type(a))
b = np.matrix('1.0 2.0;3.0 4.0')
print(type(b))
# 另一种b
b = np.arange(2,45,3).reshape(5, 3)
b = np.mat(b)
print('matrix a:')
print(a)
print('matrix b:')
print(b)
c = a*b
print('matrix c:')
print(c)
结果:
<class 'numpy.matrix'>
<class 'numpy.matrix'>
matrix a:
[[ 0 1 2 3 4]
[ 5 6 7 8 9]
[10 11 12 13 14]
[15 16 17 18 19]]
matrix b:
[[ 2 5 8]
[11 14 17]
[20 23 26]
[29 32 35]
[38 41 44]]
matrix c:
[[ 290 320 350]
[ 790 895 1000]
[1290 1470 1650]
[1790 2045 2300]]
使用循环结构计算过程:
# Program to multiply two matrices using nested loops
# 4x5 matrix
X = [[ 0 , 1 , 2 , 3 , 4],
[ 5 , 6 , 7 , 8, 9],
[10 ,11 ,12 ,13 ,14],
[15 ,16, 17 ,18, 19]]
# 5x3 matrix
Y = [[ 2 , 5 , 8],
[11, 14, 17],
[20, 23, 26],
[29,32 ,35],
[38, 41, 44]]
# result is 4x3
result = [[0,0,0],
[0,0,0],
[0,0,0],
[0,0,0]]
# iterate through rows of X
for i in range(len(X)):
# iterate through columns of Y
for j in range(len(Y[0])):
# iterate through rows of Y
for k in range(len(Y)):
result[i][j] += X[i][k] * Y[k][j]
for r in result:
print(r)
结果:
[290, 320, 350]
[790, 895, 1000]
[1290, 1470, 1650]
[1790, 2045, 2300]
后记
计算过程弄明白了,但是为什么这样算。有什么实际意义,通过一顿搜索,看到了孟岩老师的理解矩阵123,才扒开一点云雾。
链接如下:
理解矩阵(一)
理解矩阵(二)
理解矩阵(三)
- 1
- 0
-
赞助
支付宝
微信
-
分享